How to prepare for the Coding Interview?
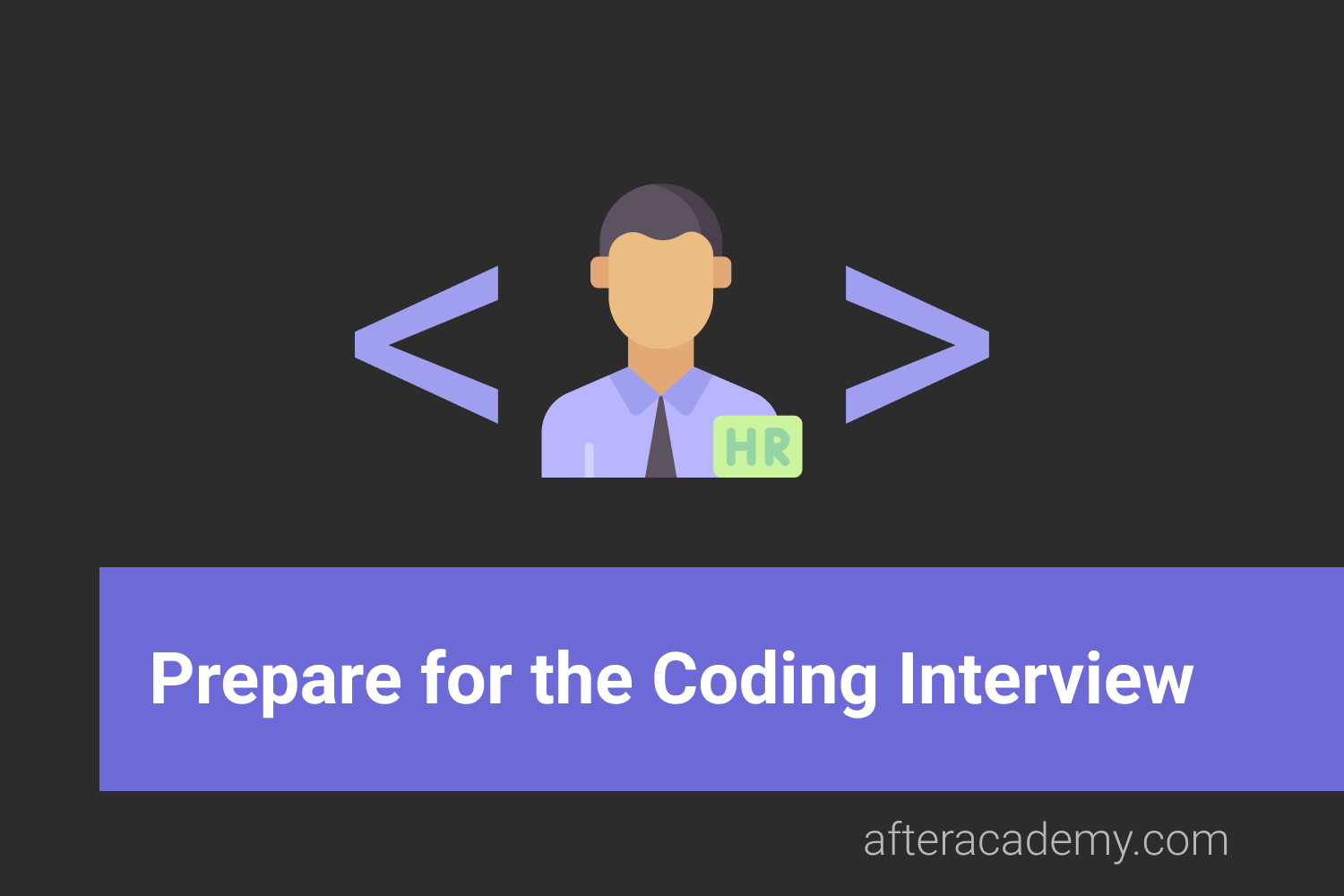
Coding interviews directly focuses on concepts and implementation of data structures and algorithms.
An interviewer tends to give you a coding question and you have been asked to solve it. There will be a discussion on the code you have written for the given problem statement and also the complexity analysis.
The interviewer may further ask you to improve the solution in terms of its number of comparison, time or space complexity. There may be follow up questions for that problem. If you are getting started with competitive programming, you should read this blog .
How to Prepare?
The preparation strategy for these interviews depends on your prior knowledge and experience of algorithms and the time left for your interview.
This blog is written by considering that you have some knowledge about data structures and have one to two months left for your interview.
Below are some important points to consider during your preparation:
- Do targeted preparation for the company you’re aiming to interview
- Use resources that are tailored to coding interview preparation
- Make cheat sheets and flashcards
- Brushup the core programming language concepts
- Do Mock interviews
- Secure as much time as you can
Targeted preparation
Different companies have different strategies for selecting the candidate and also differ in the difficulty of coding problems. It is always expected that you would encounter an entirely new problem during the interview, however, the concept would be similar to some of the problems you have practised before.
- Review popular problems and talk to your connections who may have interviewed at that company before.
- Talk to your recruiter in that company and ask them about the interview process and the things it emphasizes. That info is not often very precise, but it at least gets some things out of your way e.g. coding language, types of problems you need to focus on, etc.
Spend three to four hours every day to solve those question which has been previously asked by the company.
Resource Selection
We recommend you to follow a coherent preparation pattern to prepare for the interview while sticking with your schedule for the entire period.
- Use books like Elements of Programming Interviews, or Cracking the Coding interview.
- Use websites that have previously asked questions for those companies and practice there.
- Look for the most recent programming questions that the company is asking.
- Most of the time the recruiters share the resources to prepare from, you can also start your preparation from those resources.
Cheat sheets and Flashcards
During our preparation, we come across various new concepts and astonishing facts, storing them in a short note is worth it when you will review them just before the doomsday or you may also use them to review problems quickly later.
Revision/repetition will strengthen/deepen your understanding of core concepts. Spend about 2 hours every day for a month to strengthen your core concepts and completely cover it up.
Do mock interviews
- One or two mock interviews every week is enough, to get the first level of dust off.
- Do them with experienced engineers who routinely do interviews, instead of with friends.
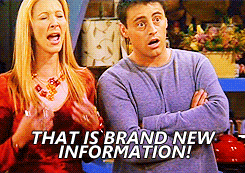
What to prepare?
There are numerous concepts of computer science that are required while solving a coding problem.
We would recommend you to must do these topics during your preparation and try to solve 15 to 20 problems on each of the below topics before your interview. Some of the topics might be difficult but you should focus more on those topics like Dynamic programming, Graphs →
- How to Analyse time and space complexity
- OOPs Concepts — Abstraction, Encapsulation, Inheritance, Polymorphism
- Iteration and two-pointer approach
- Recursion and divide and conquer
- Array and linked-list
- Stack and Queue
- Binary Tree and Binary Search Trees
- Heap and Priority Queue
- Hash Table
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Graph
- Advanced data structures Like Trie or RB Trees
Algorithms You Must Revise Before The Interview
You should complete the following concepts in the given order.
Array
- Reverse an array without affecting special characters
- All Possible Palindromic Partitions
- Count triplets with the sum smaller than a given value
- Convert an array into Zig-Zag fashion
- Pythagorean Triplet in an array
- Length of the largest subarray with contiguous elements
Searching and sorting
- Binary Search
- Quick Sort
- Merge Sort
- Order Statistics
- KMP algorithm
- Z’s algorithm
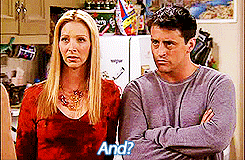
Dynamic Programming
- Longest Common Subsequence
- Longest Increasing Subsequence
- Edit Distance
- Minimum Partition
- Ways to Cover a Distance
- Longest Path In Matrix
- Subset Sum Problem
- 0–1 Knapsack Problem
Number theory and mathematical
- Sieve of Eratosthenes
- Fermat method
- Segmented Sieve
- Wilson’s Theorem
- Prime Factorisation
Graphs
- Breadth-First Search (BFS)
- Depth-First Search (DFS)
- Shortest Path from source to all vertices — Dijkstra
- Shortest Path from every vertex to every other vertex — Floyd Warshall
- Minimum Spanning tree — Prim
- Minimum Spanning tree — Kruskal
- Topological Sort
- Bridges in a graph
Conclusion
Spend as much time as you can on coding. Remember, during the interview the interviewer wants you to code the problem on a paper or a whiteboard, so be thorough with the programming language you choose to code.
Revise all the programming concepts discussed above and the tricks to keep in mind during your preparation. When you feel confident about solving coding problems then you should seriously give multiple mock interviews and ask them to point out your mistakes.
Work on your speaking skills during this period as you have to convey your thought process for the problem to the interviewer before you code. Read this blog to get a better picture of how to deal with the interviewer.
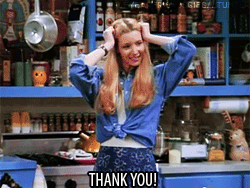
Wish you all the best for your interview.
Team AfterAcademy!