Steps of Problem Solving During Technical Interview
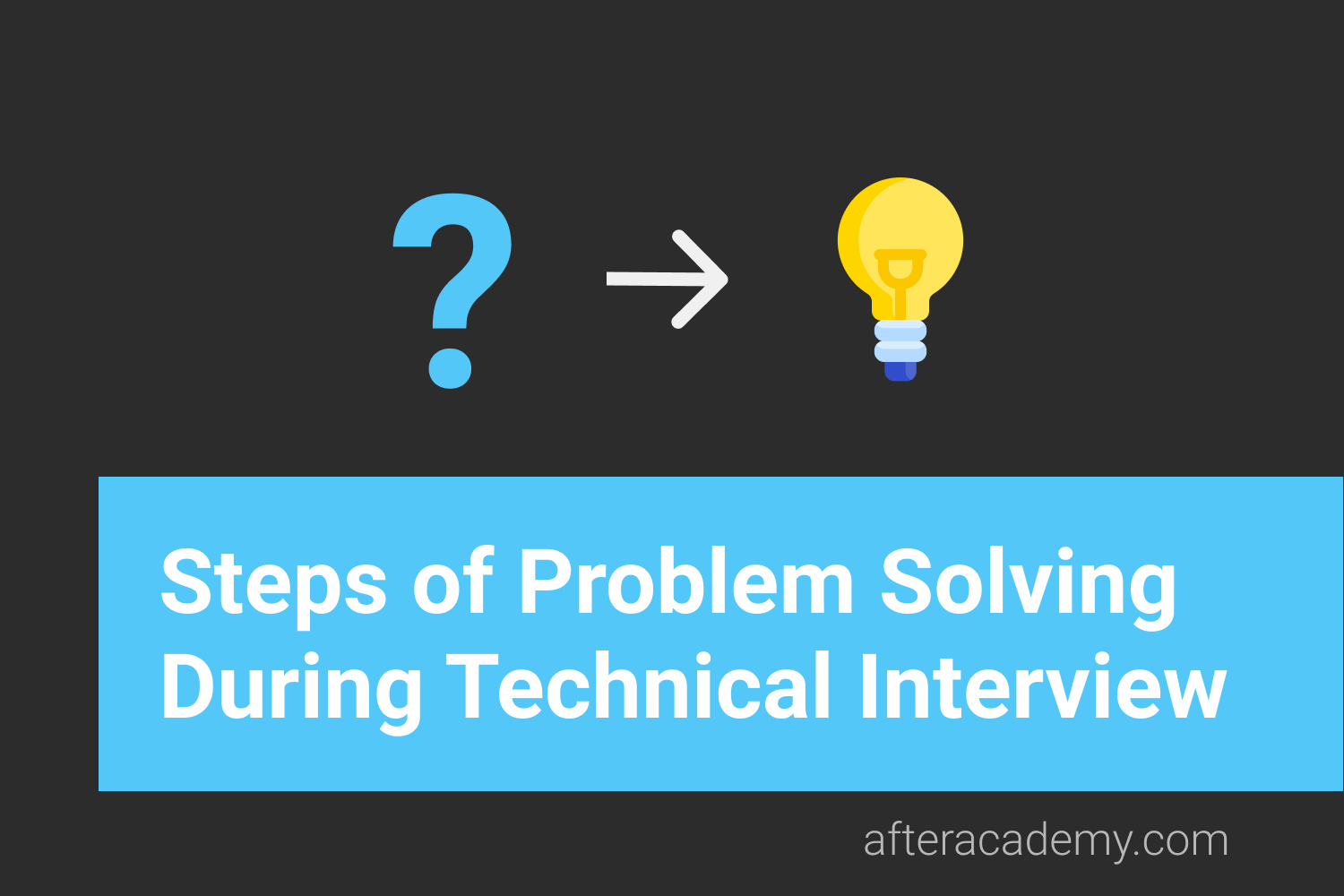
The first hurdle for a programmer to get into the software industry and land their dream job is the coding interview.
Coding Interview
The coding interview consists of Algorithms & Data structures problems. Think of these as problem-solving questions, in which the interviewer is looking to evaluate your ability to solve a problem that you haven’t seen before. One interview takes roughly 45 minutes and you are given one or two coding problems. The interviewer is expecting you to find the most optimal solution, code it and explain what you have just coded.
Before the interview, do background research. This will help you learn what kind of questions a particular interviewer would ask. Spend some time by solving similar questions. A general question is asked that, what should one do if he doesn’t know how to approach the problem right off the bat?
“Well, you won’t know what to do if it’s a good interview question”. The goal of a coding interview is to get a grasp of your coding abilities.
The absolute best candidates require very little guidance from the interviewer and lead the conversation.
These candidates will usually go through these steps by themselves with little to no prompting from the interviewer →
- Introduction
- Problem understanding
- Solution design
- Analyze
- Efficient solution
- Design code and analysis
- Code Implementation
- Test cases
- Bug resolution (scope)
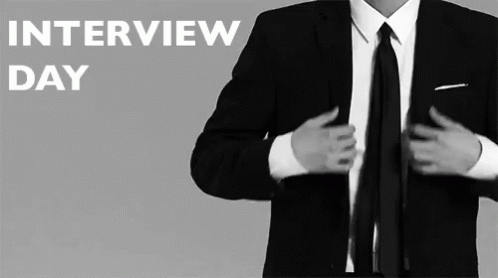
1. Introduction
Don’t try to be flattering. It’s easy to spot a fake personality. So be sure you show your true personality. Typically, he is interested in the projects or internships you have worked on before and which was the most impressive one. Now, this is your chance to shine, so don’t waste it and make a good impression of you by your introduction.
2. Problem Understanding
The interviewer will give a brief about the problem statement that you needed to solve. To find out the details about the constraints, corner cases or how you receive the data, it’s part of your job to ask for everything that you need to solve the problem. So, ask clarifying questions and read the problem statement to be sure that you got it correctly.
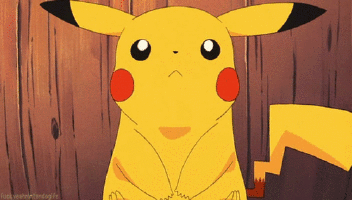
Some examples of these clarifying questions could be:
- Can we make any assumptions about the input?
- Should this be done be in place? (No additional memory)
- Try going through some custom test cases or examples and discuss the possible output for those cases.
- How big is the range of values?
- How is the input stored? If you are given a dictionary of words, is it a list of strings or a trie?
Now, Ask yourself
- Are you able to understand the question fully?
- What and how many inputs are required?
- What would be the output for those inputs?
- Do you have enough information? If not, try understanding the question again.
Too often, candidates make assumptions about the problem (like, all integers are positive, arrays are not empty). Instead, just ask the interviewer! So, it is better to be clear than to write the wrong solutions.
3. Solution Design
When a question is asked to you, do not answer right away, even if you have faced that question before. Take a little time to revise the solution you are trying to give.
Always solve the problem algorithmically first. You may start with a brute-force approach. If your algorithm is solid, coding will be easy. Communicate it to the interviewer. It is unlikely that the brute-force approach will be the one that you will be coding. At this point, the interviewer may ask, “Can we do better?”.
The interviewer will always be interested in seeing your thinking process and hearing your explanations. Most people stay quiet for three to four minutes while thinking of a solution, and that is the worst strategy possible for them.
Don’t be scared that you might start with a bad(less optimal) solution, there is time to improve it!
Finding the solution might not come naturally at once. So, when you receive the problem, try to think of the data structure that you might use. You may also list out a few potential approaches you could use to solve this problem(e.g iteration, recursion, dynamic programming, etc). After you spot the data structure, think of typical algorithms and problems that you might have seen or could relate before using that data structure.
Commonly these data structures would be helpful deriving the solution→
- Arrays, Stack, Queue
- Set, Ordered map, Hashmap
- Singly, Circular, Doubly Linked List
- Binary tree, Generic Tree, Trie, Graph
4. Analyze
Now, you have reached a solution for the problem, but that might not be the most optimal solution for the problem and so it is important for you to discuss the time-space tradeoff with the interviewer. Acknowledging the weak links in your approach demonstrates that you’re aware where potential trouble spots may arise, and offers up why you were willing to make your trade-offs. So, explaining the idea in the concept first without using too much code and it is important not to start coding right away for complex problems so that you don’t waste time writing code for the solution that doesn’t work in the end.
Now, if the interviewer asks you to code it down, then move ahead otherwise they might ask you to improve your solution complexity or intricacy.
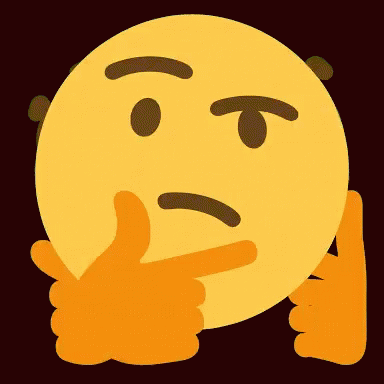
While analyzing, you may compare these points →
- Time and Space complexity.
- The number of comparisons and iteration redundancies.
- Recursive stack space or recalculations.
5. Efficient Solution
Look for repeated work and try to optimize them by potentially caching the calculated result somewhere. Reference it later, rather than computing it all over again.
Only start coding after you and your interviewer have agreed on an approach and you have been given the green light. In case you are not sure if the approach you came up with is optimal then you may ask a question similar to this to the interviewer, “I think the following approach is good, should I start coding with that?”. Just by asking, you are communicating more with the interviewer which is a good thing and you will also be sure that you are going in the right direction.
To discover an efficient solution, you may look for these points →
- Memorize the recalculations using dynamic programming.
- Two pointer approach to reduce some unnecessary calculations.
- Use of some data-structure like tries, dictionaries or balanced trees.
6. Design Code and analyse
Now we arrived at the interesting part, coding the solution. To do well in this part, you may write a pseudo code but its always better to code in the language that you must feel comfortable with.
When you start coding then it’s also about talking loud and explaining what you are coding.
The interviewer doesn’t want you to code in silence for ten minutes and then say that you have finished. Talk about it at a higher level while you are coding. It is always a good practice to use the function and variable names according to their work that makes sense. Make your code look clean and neat. No one likes messy codes that one can barely read. Be sure that your code is self-explanatory.
During analysis →
- Think of the situations how your algorithm response for different possibilities on inputs.
- Have a pseudo-code in mind for a clear thought of the solution.
- Explain it to the interviewer through a generic example.
7. Code Implementation
Start writing your solution as you explain the code and this process could be iterative in nature. So, you might write a little bit of code and you might go back to thinking a little bit about your solution and then write your code a little bit more. I recommend to always think with specific examples as you write code.
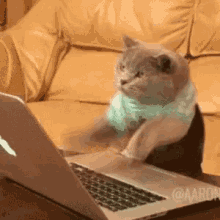
So, While designing code, make sure to have these points →
- Keep your code clean, as pen and paper could be messy.
- The names for the variables should make sense.
- The use of OOPS concept would be an add on.
- Explain what you are doing as you code in brief to the interviewer.
8. Test Cases
After coding the complete solution for the problem, try to look for the possible corner cases that might have not handled properly. Think of some possible test cases or upper limits of the input to which your code will hold good and highlight them to the interviewer.
9. Bug Resolution
Many interviewers ask to present the final code without any bugs or syntactical errors. So, before saying that you are done with the problem, take a few seconds and look over the solution with a fresh mind, whether it will work or not.
Additional pointers
For big problems, I recommend these few points that could make your code readable.
- Divide the problem into different modules or sub-problems
- Try to make independent functions for each sub-problem, you may utilize the features of OOPS too.
- Connect those sub-problems by calling them in the required order, or as necessary (Probably one function would be calling another)
In case if you don’t have any idea, what to do!
Ask for help if you’ve exhausted your train of thought. A critical factor in assessing Engineers is how they collaborate with others, and it sure beats staring at a whiteboard in silence. The interviewer won’t solve the problem for you, but they will provide hints if needed, this is done very frequently. Build on the hints, and break down your problem into small pieces. Try various solutions, and don’t be afraid of the brute force approach — you can optimize it later if needed.
Reduce nervousness
And most important, relax and show confidence throughout the interview. When you are given the problem, just try to solve it casually and dust off the fear of failure from your mind. Treat this as a situation where you are meeting new people and making some new friends, with some fun challenges thrown in the mix, not a do-or-die pressure-cooker.
If you have one bad interview round, shake it off immediately. Put it in the back of your mind and absolutely kill the others. It is very, very rare that candidates go through the entire interview process having aced every single session. There are multiple coding sessions that add to the data points from everyone you interview with.
Key points to keep in mind during the interview
- Ask your interviewer questions and actively listen to their response.
- Communicate your thought process.
- Stay calm and relax while try only focusing on the problem during the interview.
- Practice explaining the logic on a whiteboard before an interview.
So, Be yourself, show confidence and communicate well with your interviewer.
Whiteboard Interview
Have a look at our Whiteboard Interview series to find how to solve a problem during technical interviews.
Happy coding! Enjoy Algorithms.