Reverse An Array
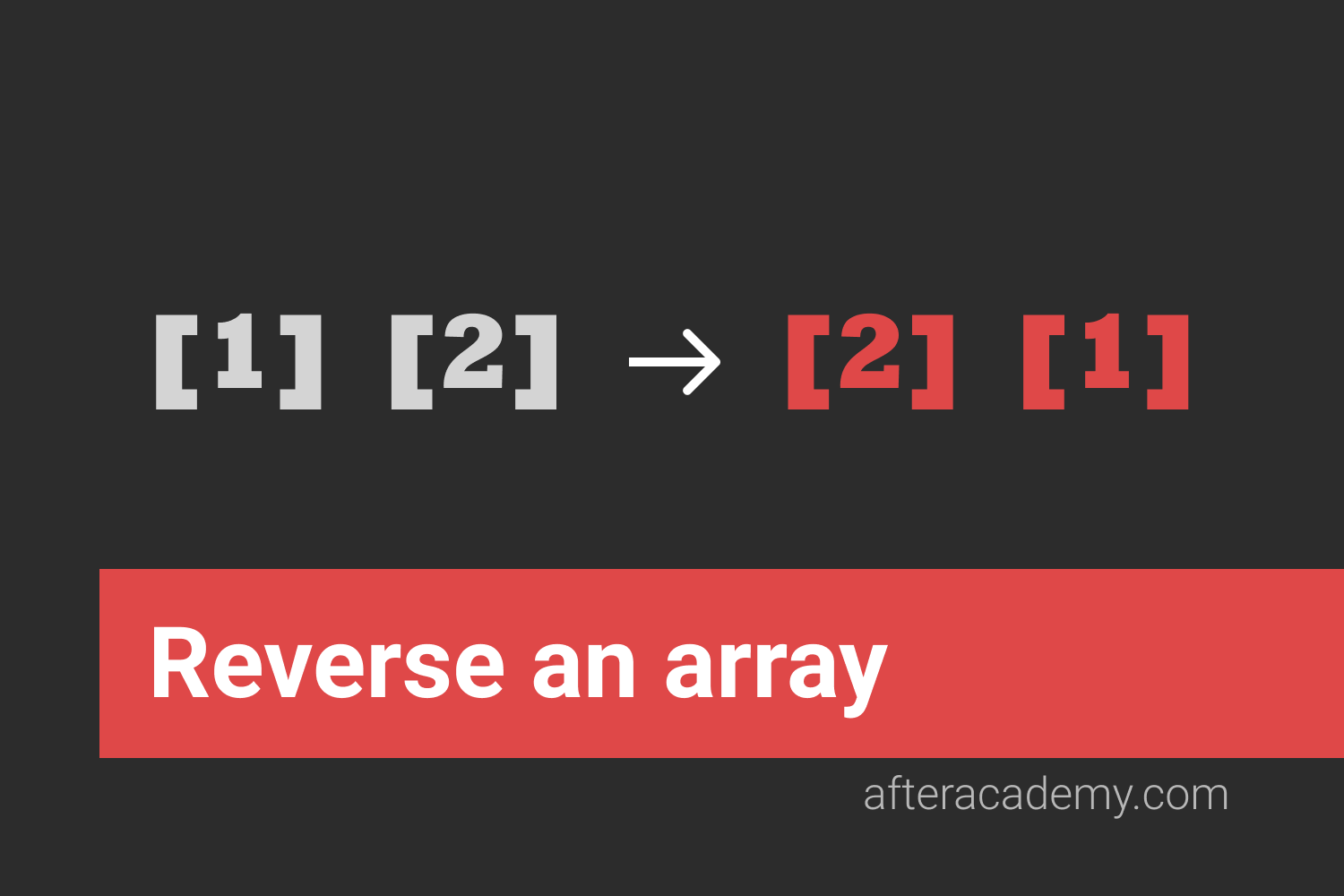
Difficulty: Easy
Unders t anding The Problem
Problem Description
Given an array, the task is to reverse the array.
Examples →
Input : arr[] = {34, 12, 23}
Output : arr[] = {23, 12, 34}
Input : arr[] = {1, 2, 3, 4, 5, 6}
Output : arr[] = {6, 5, 4, 3, 2, 1}
Solution
We will be discussing a simple approach to reverse the array in-place. In place means, we will not be using some auxiliary space.
To reverse an array, we will use the concept of swapping. Swapping is a term used for interchanging the values at two different locations with each other.
Look at the below example to get a clear understanding of the array that should be reversed.
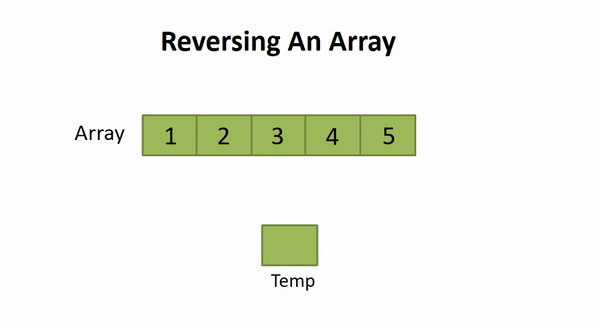
So, we can set two pointers at the start and end of the array and start iterating over it till the
. For every
(length of array)/2
, we will swap
idx
with
array[idx]
. Thus, the array will be reversed in-place.
array[length - idx]
Solution Steps
-
Place the two pointers (let
start
end
) -
Swap
arr[start]
arr[end]
-
Increment
start
end
1
-
If
start
length/2
start ≥ end
Pseudo Code
void rvereseArray(int[] arr) {
start = 0
end = arr.length - 1
while (start < end) {
// swap arr[start] and arr[end]
int temp = arr[start]
arr[start] = arr[end]
arr[end] = temp
start = start + 1
end = end - 1
}
}
Complexity Analysis
Time Complexity: O(n)
Space Complexity: O(1)
Critical Ideas to Think
-
The loop will run only till
length/2
O(n)
O(n/2)
- How did we perform swapping?
-
Why did we iterate until
start < end
start < (length of array)/2
-
What will happen if the while condition would be
start < length of array
start < end
Suggested Problems To Solve
- Write a program to reverse digits of a number
- Program to reverse a string
- Program to reverse words in a given string.
- Program to reverse the rows in a 2d Array
- Program to Reverse Bits of a Number