Gas Station Problem
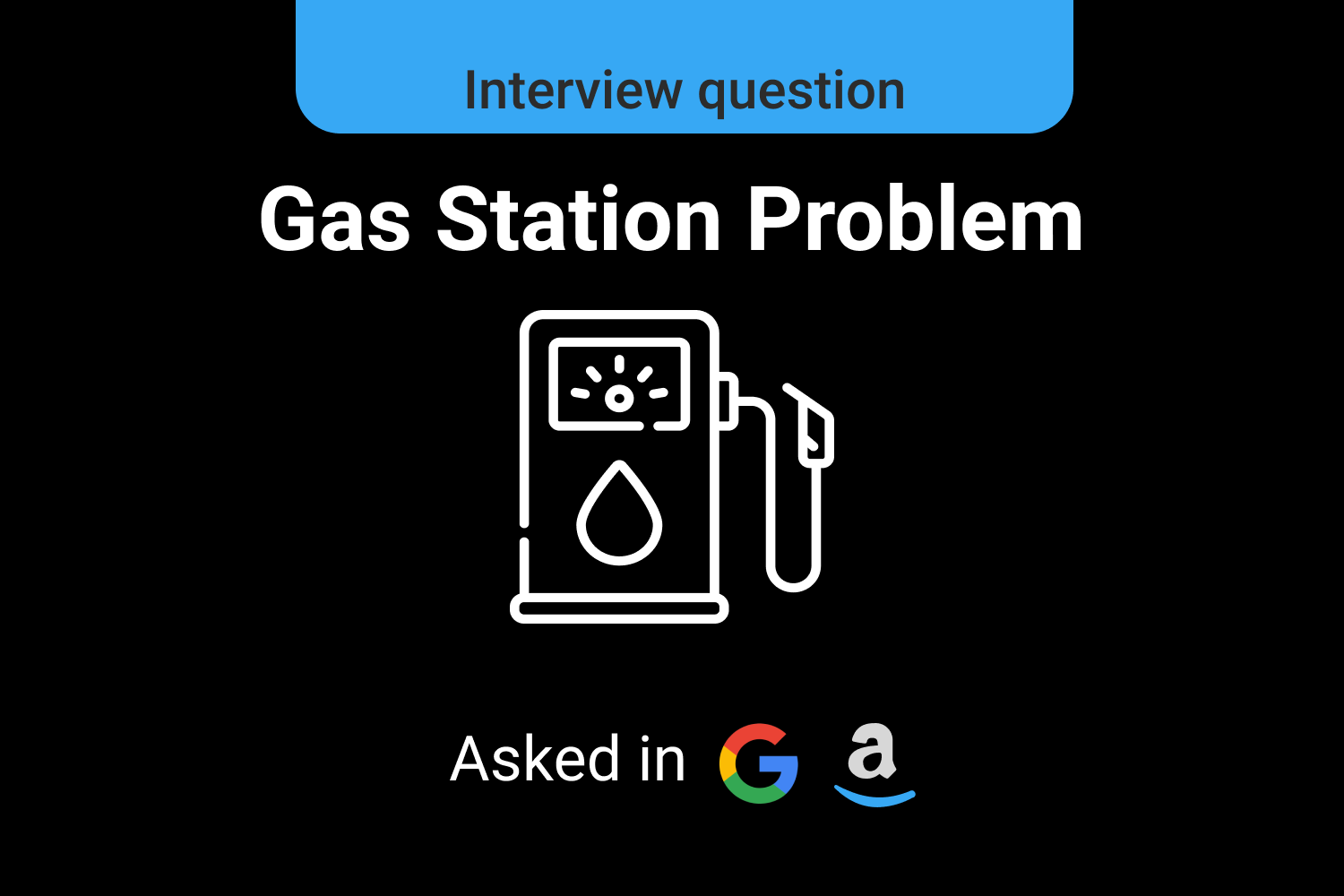
Difficulty: Medium
Asked in: Amazon, Google
Understanding The Problem
Problem Description
There are
N
gas stations along a circular route, where the amount of gas at station
i
is
. You have a car with an unlimited gas tank and it costs
arr[i]
of gas to travel from station
cost[i]
to its next station
i
(
. At the beginning of the journey, the tank is empty at one of the gas stations.
i+1
)
Return the minimum starting gas station’s index if you need to travel around the circuit once, otherwise return -1.
Problem Note
-
Completing the circuit means starting at
i
i
- Both input arrays are non-empty and have the same length.
- Each element in the input arrays is a non-negative integer.
Example 1
Input:
arr[] = [1,2,3,4,5]
cost[] = [3,4,5,1,2]
Output: 3
Explanation:
Start at station 3 (index 3) and fill up with 4 unit of gas. Gas available in Tank = 0 + 4 = 4
Travel to station 4.Gas available in tank = 4 - 1 + 5 = 8
Travel to station 0.Gas available in tank = 8 - 2 + 1 = 7
Travel to station 1.Gas available in tank = 7 - 3 + 2 = 6
Travel to station 2.Gas available in tank = 6 - 4 + 3 = 5
Travel to station 3.The cost is 5.Your gas is just enough to travel back to station 3.Therefore, return 3 as the starting index.
Example 2
Input:
arr[] = [2,3,4]
cost[] = [3,4,3]
Output: -1
Explanation:
You can't start at station 0 or 1, as there is not enough gas to travel to the next station.
Let's start at station 2 and fill up with 4 unit of gas. Gas available in tank = 0 + 4 = 4
Travel to station 0.Gas available in tank = 4 - 3 + 2 = 3
Travel to station 1.Gas available in tank = 3 - 3 + 3 = 3
You cannot travel back to station 2, as it requires 4 unit of gas but you only have 3.Therefore, you can't travel around the circuit once no matter where you start.
Solutions
We will be discussing a brute force and a greedy solution for the problem
- Brute Force Solution: Check if you can cover all the gas stations starting from any station. If that’s not possible, repeat it by choosing another starting station.
- Greedy Solution : Optimize the brute force by concluding that if you cannot cover station B from station A, then any station between them cannot lead to an answer.
1. Brute Force Solution
For each station
choose it as a starting point, and try to visit every other station and maintain a
i
to that will tell about the possibility of visiting the next station, if not then choose some other starting station and try to visit until you have reached the starting station.
fuel
Solution Steps
- Choose the station as a starting point.
- Simulate the road trip to see if the following stations are reachable.
Pseudo Code
int canCompleteCircuit(int[] gas, int[] cost) {
n = gas.size()
for (int i = 0 to i < n) {
fuel = gas[i]
bool isAmple = true // determine a valid starting point
for (int j = 0 to j < n) {
currStation = (i + j) % n
nextStation = (currStation + 1) % n
fuel = fuel - cost[currStation]
// not reachable from currStation to nextStation
if (fuel < 0) {
isAmple = false
break
}
// refuel in nextStation
fuel = fuel + gas[nextStation]
}
if (isAmple) {
return i
}
}
return -1
}
Complexity Analysis
Time Complexity: O(n²)
Space Complexity: O(1)
Critical Ideas To Think
- How did we cycle to the first station from the last station while picking some intermediate station as the starting point?
- If the fuel is empty in the middle of two adjacent stations, then what did we do?
-
What does
isAmple
-
How did we check, if it is possible or not to move from
currStation
nextStation
2. Greedy Solution
The problem enables you to have these two ideas:
- If the car starts at A and can not reach B. Any station between A and B can not reach B.(B is the first station that A can not reach.)
- If the total number of gas is bigger than the total number of cost. There must be a solution.
The proof of the above two ideas:
- In any station between A and B, let’s say C. C will have gas left in our tank, if we go from A to that station. We can’t reach B from A with some gas(maybe 0) left in the tank in C, so we can’t reach B from C with an empty tank.
- If the gas is more than the cost in total, there must be some stations we have enough gas to go through them. Let’s say they are green stations. So the other stations are red . The adjacent stations with the same color can be joined together as one. Then there must be a red station that can be joined into a precedent green station unless there isn’t any red station because the total gas is more than the total cost. In other words, all of the stations will join into a green station at last.
This Concludes:
- If the sum of gas is more than the sum of cost, then there must be a solution. And the question guaranteed that the solution is unique(The first one you found will the right one).
- The tank should never be negative, so restart whenever there is a negative number.
Solution Steps
-
Create a
start
-
For each station
i
gas[i]
cost[i]
-
If at any point the tank is
< 0
- At last, check if the total fuel available at the gas stations is greater than the total fuel burnt during the travel.
-
Return the
start
Pseudo Code
int canCompleteCircuit(int[] gas, int[] cost) {
start = 0
total = 0
tank = 0
for( i = 0 to i < gas.size() ) {
tank = tank + gas[i] - cost[i]
if(tank < 0) {
start = i+1
total= total + tank
tank=0
}
}
if(total + tank < 0) {
return -1
} else {
return start
}
}
Complexity Analysis
Time Complexity: O(n)
Space Complexity: O(1)
Critical Ideas To Think
-
Whenever
tank
< 0
start
-
If the
tank
< 0
0
. Why? -
Why did we return -1 if
total + tank < 0
- How did we ensure that whether or not a solution exists for the input?
Comparison Of Different Approaches
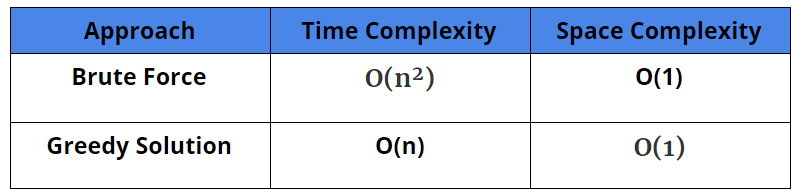
Suggested Problems To Solve
- Check if a given sequence of moves for a robot is circular or not
- Circular Queue Implementation
- Sorted insert for circular linked list
- Minimum circular rotations to obtain a given numeric string by avoiding a set of given strings
- Check if a linked list is Circular Linked List
- Find four elements that sum to a given value | Set 2
If you have any more approaches or you find an error/bug in the above solutions, please comment down below.
Happy Coding! Enjoy Algorithms!